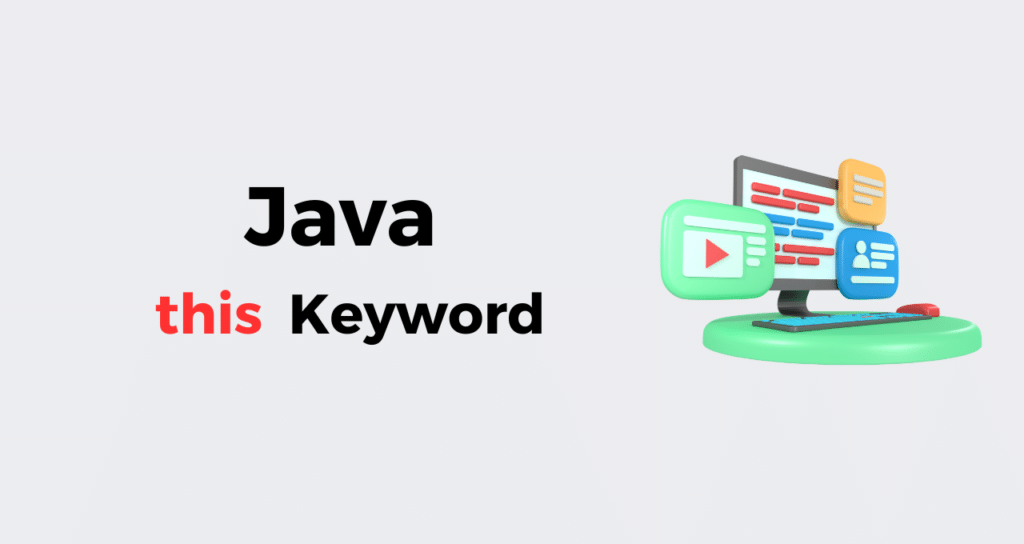
Understanding the this
Keyword in Java
What is the this
Keyword in Java?
In Java, the this
keyword is a reference variable that refers to the current object within a method or constructor. It helps in differentiating between instance variables and local variables when they have the same name.
Why Use this
Keyword in Java?
The this
keyword in Java enhances code readability and removes ambiguity by clearly referring to instance variables. It is widely used for:
Referring to instance variables of the current class
Calling or invoking the current class constructor
Passing as an argument in method and constructor calls
Returning the current class instance
How this
Keyword Works: Example & Explanation:
Consider the following Java program:
javaclass Account {
int a;
int b;
public void setData(int a, int b) {
this.a = a; // Using 'this' to differentiate instance and local variables
this.b = b;
}
public void showData() {
System.out.println("Value of A = " + a);
System.out.println("Value of B = " + b);
}
public static void main(String args[]) {
Account obj = new Account();
obj.setData(2, 3);
obj.showData();
}
}
Understanding the this Keyword in Java Expected Output:
javaValue of A = 2
Value of B = 3
Without the this
keyword, the compiler gets confused between instance variables and local parameters, leading to incorrect values. Adding this
ensures proper variable assignment.
Understanding the this Keyword in Java Use Cases of this
Keyword in Java:-
1. Accessing Instance Variables:
When a constructor or method parameter has the same name as an instance variable, this
helps to access the instance variable.
javaclass Example {
int num;
Example(int num) {
this.num = num; // 'this' refers to the instance variable
}
}
2. Calling Another Constructor in the Same Class:
The this()
keyword can be used to invoke another constructor within the same class.
javaclass Test {
int a, b;
Test() {
this(5, 10); // Calls parameterized constructor
}
Test(int a, int b) {
this.a = a;
this.b = b;
}
}
3. Passing as an Argument in Method Calls:
this
can be passed as an argument to other methods for referencing the current object.
javaclass Demo {
void show(Demo obj) {
System.out.println("Method is called");
}
void display() {
show(this); // Passing the current object
}
}
4. Returning the Current Object:
this
can be used to return the current object in method chaining.
javaclass Example {
Example get() {
return this; // Returning the current instance
}
}
Java Learning Guide:-
If you’re searching for:
Java
this
keyword explanation. When to use
this
in Java.
this
keyword examples in Java. Java best practices for constructors and methods.
This guide provides a detailed and friendly breakdown to help beginners and professionals understand the this
keyword in Java.
Conclusion:-
The this
keyword is an essential part of Java programming that helps manage object references efficiently. It ensures clarity when dealing with instance and local variables, constructor calls, and method arguments. Understanding its use cases enhances code maintainability and reduces errors.
Learn Java with practical examples.
Enhance coding efficiency with the
this
keyword. Improve Java skills for interviews and real-world applications.
For more Java tutorials and advanced coding techniques, keep exploring and coding! .