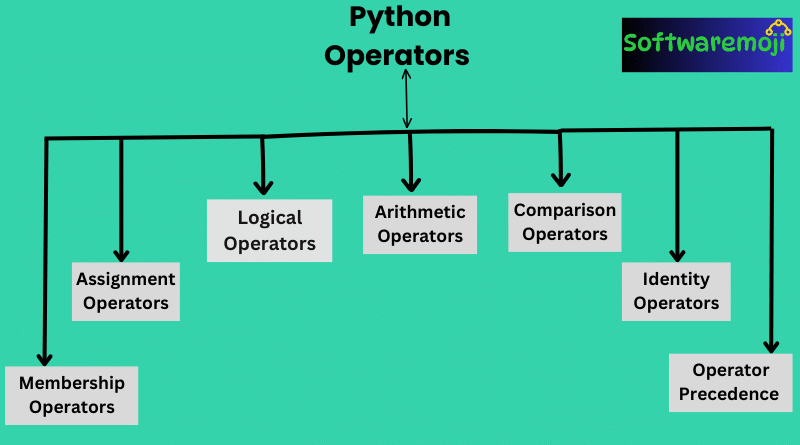
👉Python Operators Explained
👉Python Operators Overview
Python Operators Explained: Python operators are special symbols or keywords used to perform operations on variables and values. They are crucial for performing arithmetic, logical, and comparison operations in Python programs. Let’s explore the key types of operators in Python.
👉Logical Operators in Python
👉Python Operators Explained:
Logical operators are used to determine the logic between conditions. The result is either True or False. There are three primary logical operators in Python:
- AND Operator (and): Returns True if both conditions are true.
- OR Operator (or): Returns True if at least one condition is true.
- NOT Operator (not): Reverses the result, returning True if the condition is false.
👉Example:
a = True
b = False
print(‘a and b is’, a and b) # False
print(‘a or b is’, a or b) # True
print(‘not a is’, not a) # False
👉Arithmetic Operators in Python
Python Operators Explained: Arithmetic operators are used to perform mathematical operations such as addition, subtraction, multiplication, etc.
👉Examples of Arithmetic Operators:
x = 4
y = 5
print(x + y) # Addition: 9
print(x – y) # Subtraction: -1
print(x * y) # Multiplication: 20
print(x / y) # Division: 0.8
print(x % y) # Modulus: 4
👉Comparison Operators in Python
Comparison operators compare two values and return either True or False. Common operators include:
- == (Equal)
- != (Not equal)
- > (Greater than)
- < (Less than)
- >= (Greater than or equal to)
- <= (Less than or equal to)
👉Example:
x = 4
y = 5
print(‘x > y is’, x > y) # False
👉Assignment Operators in Python
Assignment operators are used to assign values to variables. Common assignment operators include:
- = (Assign)
- += (Add and assign)
- -= (Subtract and assign)
- *= (Multiply and assign)
- /= (Divide and assign)
👉Example:
num1 = 4
num2 = 5
num1 += num2
print(‘Result:’, num1) # 9
👉Python Operators Explained Membership Operators in Python
Membership operators are used to check if a value exists in a sequence such as a list, tuple, or string.
- in: Returns True if the value is present.
- not in: Returns True if the value is not present.
👉Example:
list_values = [1, 2, 3, 4, 5]
print(4 in list_values) # True
print(8 not in list_values) # True
👉Identity Operators in Python
Identity operators compare the memory locations of two objects.
- is: Returns True if both variables are the same object.
- is not: Returns True if they are not the same object.
👉Example:
x = 20
y = 20
print(x is y) # True
y = 30
print(x is not y) # True
👉Operator Precedence in Python
Operator precedence determines the order in which operators are evaluated in an expression. Higher precedence operators are evaluated first.
👉Example:
v = 4
w = 5
x = 8
y = 2
z = (v + w) * x / y # 36.0
print(z)
👉Summary
Python operators are essential for writing efficient code. Understanding the behavior of Logical, Arithmetic, Comparison, Membership, and Identity operators, along with operator precedence, is key to mastering Python programming. Practicing these operators with examples will enhance your coding skills.