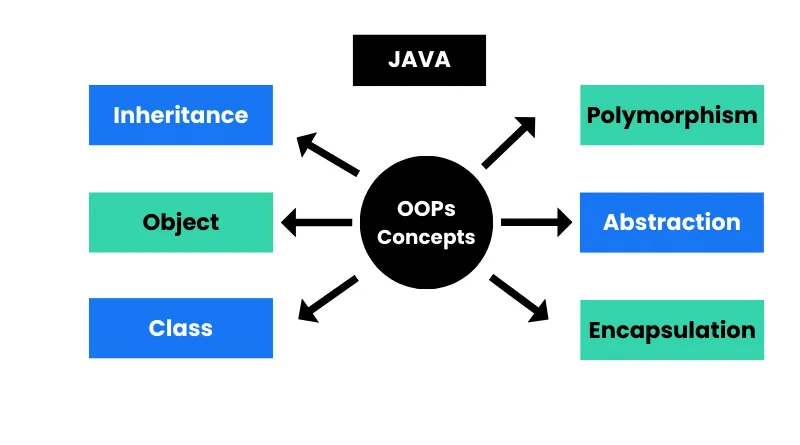
OOPs Concepts in Java:
✅What is Object-Oriented Programming (OOPs)?
Object-Oriented Programming (OOPs) is a programming paradigm that focuses on objects rather than functions and procedures. It enhances code reusability, scalability, and maintainability by structuring code into objects, which represent real-world entities. Java is one of the most popular OOP-based languages.
👉Tutorial-1:-Abstraction in Java.
👉Tutorial-2:-Encapsulation in Java.
✅Key OOPs Concepts in Java with Examples:-
1. Class:
A class in Java is a blueprint for creating objects. It defines properties (attributes) and behaviors (methods).
🔹 Example:
javaclass Car {
String brand;
int speed;
void accelerate() {
System.out.println(brand + " is accelerating.");
}
}
Here, Car
is a class with attributes brand
and speed
and a method accelerate()
.
2. Object:
An object is an instance of a class. It holds actual data and invokes methods.
🔹 Example:
javapublic class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.brand = "BMW";
myCar.accelerate();
}
}
myCar
is an object of the Car
class.
3. Inheritance:
Inheritance allows a class (child) to acquire properties and behaviors of another class (parent).
🔹 Example:
javaclass
Vehicle {
int speed = 60;
}
class Car extends Vehicle {
void showSpeed() {
System.out.println("Speed: " + speed);
}
}
Here, Car
inherits the speed
property from Vehicle
.
4. Polymorphism:
Polymorphism enables a method to have multiple forms (method overloading and overriding).
🔹 Example:
javaclass Shape {
void draw() {
System.out.println("Drawing a shape");
}
}
class Circle extends Shape {
void draw() {
System.out.println("Drawing a circle");
}
}
Here, draw()
behaves differently in Shape
and Circle
classes.
5. Abstraction:
Abstraction hides implementation details and shows only relevant information.
🔹 Example:
javaabstract class Animal {
abstract void makeSound();
}
class Dog extends Animal {
void makeSound() {
System.out.println("Barking");
}
}
Here, Animal
is an abstract class with an abstract method makeSound()
, implemented in Dog
.
6. Encapsulation:
Encapsulation protects data by restricting direct access using private variables and public methods.
🔹 Example:
javaclass BankAccount {
private double balance;
public void deposit(double amount) {
if (amount > 0) balance += amount;
}
public double getBalance() {
return balance;
}
}
Here, balance
is private, ensuring data security.
7.Association, Aggregation & Composition:
- Association: Defines relationships between two independent objects (e.g., Teacher–Student). Aggregation: One object contains another, but the child can exist independently (e.g., Department–Professor).
- Composition: A stronger form of Aggregation, where the child object cannot exist without the parent (e.g., House–Room).
✅Benefits of OOP in Java:-
✔️Enhances code reusability.
✔️ Improves security using encapsulation.
✔️ Facilitates scalability.
✔️ Helps in modular programming.
✅Conclusion:-
Understanding OOPs concepts in Java is crucial for writing efficient and maintainable code. By using classes, objects, inheritance, and polymorphism, Java provides a structured approach to application development.
🔹 Related Topics:
- Java Design Patterns.
- Best Practices for OOP in Java.
- Difference Between Java and Python in OOP.
Would you like a deeper explanation of any specific topic? 🚀