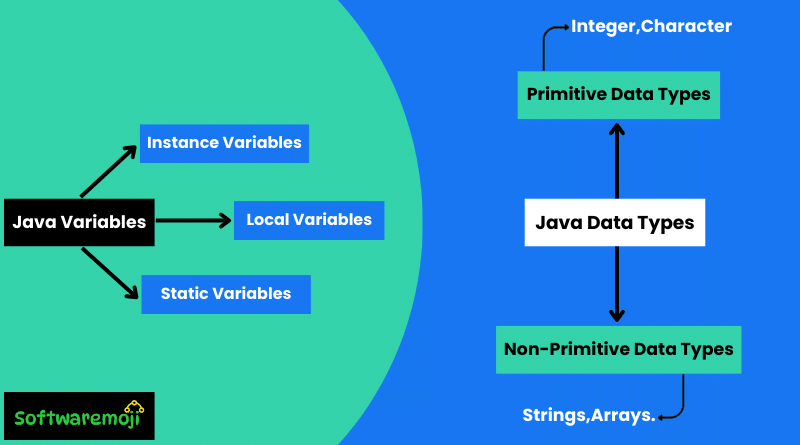
✔️Java Variables and Data Types:
Java variables and data types form the foundation of every Java program, making them essential for beginners to master.
Understanding Java data types helps developers write memory-efficient and error-free code.
Variables in Java are containers that store data values, and choosing the right data type is key to performance.
Java supports both primitive data types (like int, float, char) and non-primitive types (like String and Arrays).
👉Tutorial-1:Classes and Objects in Java.
👉Tutorial-2:Java Arrays Has Wonderful Opportunity-2025
👉Tutorial-3:Array of Objects in Java
👉Tutorial-4:Array List in Java-2025
✔️What is a Variable in Java?
A variable in Java is a reserved memory location to store values during program execution. Each variable has a specific data type that determines what kind of data it can hold.
There are three main types of variables in Java:
1.Local Variables – Declared inside a method or block.
2.Instance Variables – Defined inside a class but outside any method, and belongs to an instance of the class.
3.Static Variables – Declared using the static
keyword and shared among all instances of the class.
✔️How to Declare and Initialize Variables in Java?
A variable declaration in Java requires specifying the data type and assigning it a unique name.
Example of Variable Declaration:
javaint a, b, c;
float pi;
double d;
char letter;
Java Variables and Data Types Example of Variable Initialization:
javapi = 3.14f;
d = 20.22d;
letter = 'A';
Combined Declaration and Initialization:
javaCopyEditint x = 5, y = 10;
float pi = 3.14f;
double amount = 1000.50;
char grade = 'A';
✔️Java Variables and Data Types Types of Variables in Java with Examples:-
javaclass Example {
static int staticVar = 100; // Static Variable
int instanceVar = 50; // Instance Variable
void display() {
int localVar = 10; // Local Variable
System.out.println("Local Variable: " + localVar);
}
}
✔️Java Data Types:-
In Java, data types specify the size and type of values that can be stored in variables.
✔️Types of Data Types in Java:-
- Primitive Data Types – Includes integer, character, boolean, and floating-point numbers.
- Non-Primitive Data Types – Includes Strings, Arrays, Classes, and Interfaces.
✔️Java Variables and Data Types Primitive Data Types:-
Data Type | Default Value | Size | Description |
---|---|---|---|
byte | 0 | 1 byte | Stores whole numbers (-128 to 127) |
short | 0 | 2 bytes | Stores whole numbers (-32,768 to 32,767) |
int | 0 | 4 bytes | Stores whole numbers (-2^31 to 2^31-1) |
long | 0L | 8 bytes | Stores large whole numbers (-2^63 to 2^63-1) |
float | 0.0f | 4 bytes | Stores fractional numbers (6-7 decimal digits) |
double | 0.0d | 8 bytes | Stores large fractional numbers (15 decimal digits) |
char | ‘\u0000’ | 2 bytes | Stores a single character |
boolean | false | 1 bit | Stores true or false |
✔️Type Conversion & Type Casting in Java:-
Java Variables and Data Types .Java allows converting one data type into another either implicitly (automatic) or explicitly (type casting).
✔️Implicit Type Conversion (Widening):-
- When a smaller data type is assigned to a larger data type, Java automatically converts it.
javaint num = 10;
double newNum = num; // Implicit conversion from int to double
System.out.println(newNum); // Output: 10.0
✔️Explicit Type Casting (Narrowing):-
- When a larger data type is assigned to a smaller data type, explicit casting is required.
javadouble value = 99.99;
int newValue = (int) value; // Explicit conversion from double to int
System.out.println(newValue); // Output: 99
✔️Best Practices for Using Variables in Java:-
- Always declare variables with meaningful names to improve code readability.
- Use constants (
final
keyword) for values that should not change. - Optimize memory usage by choosing the right data type for each variable.
- Follow Java naming conventions (camelCase for variables, UPPER_CASE for constants).
- Use
static
variables carefully to avoid unexpected behavior in multi-threaded applications.
✔️Conclusion:-
Understanding Java variables and data types is essential for writing efficient and optimized Java programs. By following best practices and using the right data types, you can create more readable and performant code.
For more Java tutorials, follow our blog and stay updated! 🚀.