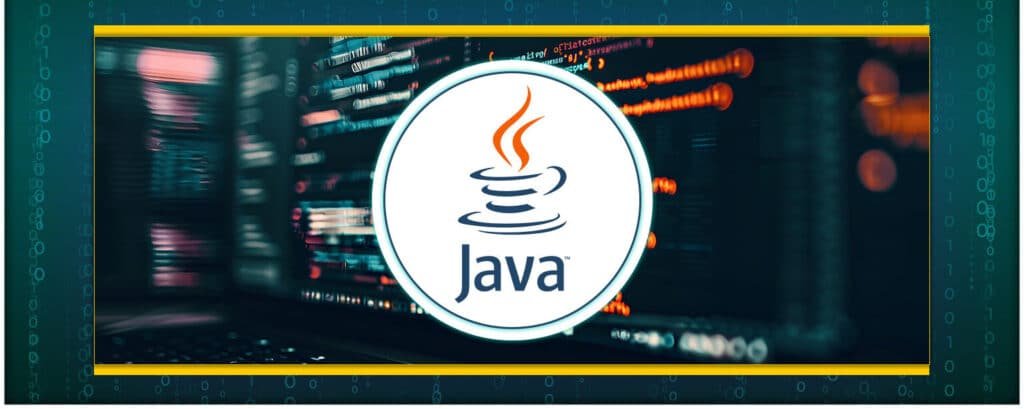
Java Hello World
If you’re new to Java programming, the Hello World program is the perfect starting point. This guide will walk you through writing, compiling, and running your first Java program efficiently.
➡️Why Learn Java?
Java is one of the most popular programming languages used for web development, mobile applications (Android), enterprise software, and more. Learning Java sets a strong foundation for building robust applications.
➡️To Running a Java Program:-
1. Install Java Development Kit (JDK):
To run Java programs, you need to install the Java SE Development Kit (JDK). Follow these steps:
- Download the latest JDK version from the https://www.oracle.com/in/
- Install and set up environment variables for Java.
2.Choose a Text Editor or IDE:
You can write Java programs in any text editor like Notepad, VS Code, or IntelliJ IDEA. For beginners, Notepad or an online Java compiler is a simple choice.
3. Write Your First Java Program:
Copy and paste the following code into your editor:
javaclass HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
4.Save and Compile the Java Program:
- Save the file as HelloWorld.java (file name must match the class name).
- Open the Command Prompt (cmd) and navigate to the folder where the file is saved.
- Compile the program using:
nginxjavac HelloWorld.java
- If there are no errors, a HelloWorld.class file will be created.
5. Run the Java Program:
To execute the program, type:
nginxjava Hello World
You will see the output:
Hello, World!
➡️Understanding Java Command Line Arguments:-
Java allows passing arguments to programs via the command line. Example:
javaclass Demo {
public static void main(String args[]) {
System.out.println("Argument 1 = " + args[0]);
System.out.println("Argument 2 = " + args[1]);
}
}
Run the program using:
mathematicajava Demo Apple Orange
Output:
mathematicaArgument 1 = Apple
Argument 2 = Orange
➡️Best Practices for Java Beginners:
✅ Always save your file with the .java extension.
✅ Java is case-sensitive, so be consistent in naming conventions.
✅ Use an IDE like Eclipse or IntelliJ IDEA for faster development.
➡️Final Thoughts:-
Java is a versatile language widely used in software development. Mastering basic syntax, compiling, and running programs is the first step toward becoming a proficient Java developer.
For more Java tutorials, stay updated with the latest Java coding practices, frameworks, and best development tools. 🚀