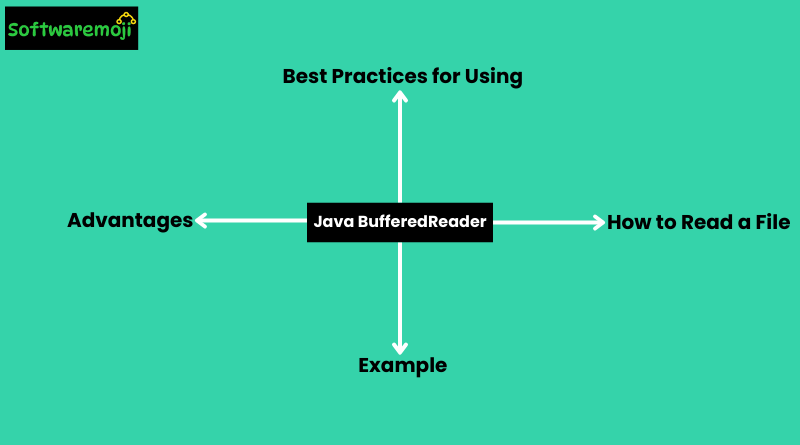
🔥Java BufferedReader:
🏹Java BufferedReader is used to read text from an input stream efficiently.
🏹BufferedReader reduces the number of I/O operations by buffering input.
🏹It belongs to the java.io
package in Java.
🏹BufferedReader is commonly used to read data line-by-line in Java.
🏹BufferedReader supports readLine()
method to read input line-by-line.
🏹It is faster than FileReader for reading large text files in Java.
🏹BufferedReader must be closed after use to avoid memory leaks.
🏹You can wrap a FileReader with BufferedReader for efficient file reading.
🏹Java BufferedReader is ideal for reading console input or file input.
🏹BufferedReader helps in reading character-based streams efficiently.
🔥How to Read a File Using Java BufferedReader?
Reading a file in Java is a common requirement in various applications. Java provides multiple ways to read files efficiently, and one of the most commonly used classes for this purpose is BufferedReader, available in the java.io
package.
Java BufferedReader
is a powerful class used to read text from input streams efficiently. It belongs to the java.io
package and buffers characters to provide fast reading of characters, arrays, or lines. It’s commonly used when reading data from files or user input via the console. By reducing the number of I/O operations, it significantly improves performance. Developers use methods like readLine()
to read text line-by-line. To use it, wrap a FileReader
or InputStreamReader
inside a BufferedReader
. This class is essential for file handling, data processing, and is SEO-relevant for Java beginners, file I/O, and efficient stream reading.
🔥What is BufferedReader in Java?
BufferedReader
is a Java class designed for reading text from an input stream, such as a file. It buffers characters to improve efficiency and can read data in the form of characters, arrays, or lines. Using BufferedReader
helps reduce the number of input operations, making file reading faster and more efficient.
- It supports reading multiple lines using loops in Java programs.
- Java developers use BufferedReader for performance-critical I/O tasks.
- BufferedReader is suitable for handling large text data in Java.
- BufferedReader throws IOException which must be handled properly.
- It is often used in Java programs requiring fast data reading.
🔥Advantages of Using BufferedReader:
✔ Efficient Performance: Reduces disk I/O operations.
✔ Reads Data Line by Line: Makes it easy to process text files.
✔ Supports Large Files: Handles large datasets without excessive memory usage.
🔥Example: Reading a File Using BufferedReader in Java:
Here’s a simple example demonstrating how to read a file using BufferedReader
:
javaimport java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
String filePath = "D:\\DukesDiary.txt"; // File path
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line); // Print each line
}
} catch (IOException e) {
e.printStackTrace(); // Handle exceptions
}
}
}
🔥Best Practices for Using BufferedReader in Java:
- Use Try-with-Resources: Ensures that resources like
BufferedReader
are closed automatically. - Check File Path: Ensure the file exists before reading to avoid
FileNotFoundException
. - Handle Exceptions Properly: Use
try-catch
blocks for error handling. - Optimize for Large Files: Use
BufferedReader
instead ofScanner
for better performance.
It supports reading multiple lines using loops in Java programs.
Java developers use BufferedReader for performance-critical I/O tasks.
BufferedReader is suitable for handling large text data in Java.
BufferedReader throws IOException which must be handled properly.
It is often used in Java programs requiring fast data reading.
🔥Conclusion:
Using BufferedReader
in Java is one of the best ways to read files efficiently. It offers faster performance, better memory management, and ease of use for reading large text files. If you’re dealing with large files, BufferedReader
is a must-use class for optimal file handling.
🚀 Looking for more Java tutorials? Stay updated with the latest Java coding tips!