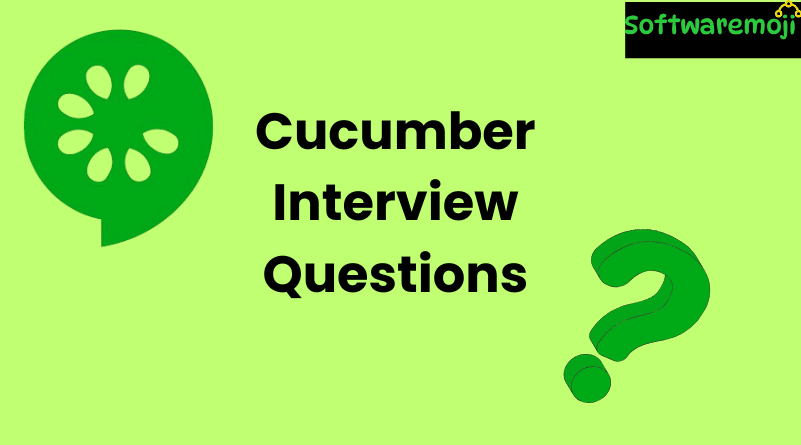
👉Cucumber Interview Questions and Answers
Introduction
Cucumber Interview Questions: Are you preparing for a Cucumber interview in 2025? Whether you’re a fresher or an experienced automation tester, these Cucumber interview questions and answers will help you ace your interview with confidence.
This guide covers all important concepts including Cucumber basics, Gherkin syntax, step definitions, BDD principles, and its integration with Selenium.
👉 Cucumber Interview Questions and Answers for Freshers
1. What is Cucumber? What are its advantages?
Cucumber Interview Questions: Cucumber is a tool used to execute functional test cases written in plain text using the Gherkin language. It was initially written in Ruby.
Advantages:
- Non-technical stakeholders can contribute to test cases.
- Focuses on the end-user behavior.
- High code reusability.
- Supports BDD approach.
2. What are the two files required to execute a Cucumber scenario?
Cucumber Interview Questions: To run a Cucumber test, you need:
- A Feature File (contains the test scenario written in Gherkin)
- A Step Definition File (contains the Ruby methods corresponding to each step)
3. What is the use of the Background keyword in Cucumber?
The Background keyword allows you to define common preconditions for all scenarios in a feature file. It’s helpful when the same Given steps are repeated across multiple scenarios.
4. Give an example of a BDD scenario in plain text.
gherkin
Feature: Visit XYZ page in abc.com
Scenario: Navigate to XYZ page
Given I am on abc.com
When I click on XYZ page
Then I should see ABC page
5. What is a Scenario Outline in a Feature File?
A Scenario Outline allows you to run the same scenario with different sets of input data, defined in a tabular format using the Examples keyword.
6. What is a Step Definition in Cucumber?
A Step Definition maps each step in a feature file to actual Ruby code. It’s where the test logic is implemented.
7. Give an example of a step definition using the Given keyword.
ruby
Given(/^I am on www.yahoo.com$/) do
browser.goto(“http://www.yahoo.com”)
end
8. What is the difference between JBehave and Cucumber?
Feature | JBehave | Cucumber |
Language Base | Java | Ruby |
Structure | Stories | Features |
Syntax | Custom DSL | Gherkin |
9. What is a Test Harness in Cucumber?
A Test Harness in Cucumber sets up and manages:
- Test environment setup
- Scenario execution
- Cleanup processes
It ensures modularity and code reusability in large test suites.
10. When to use RSpec vs Cucumber?
- RSpec is used for Unit Testing
- Cucumber is used for Behavior-Driven Development (BDD)
Suitable for System and Integration testing
👉 Cucumber Interview Questions for Experienced
11. What language is used for writing scenarios in Cucumber?
Gherkin language is used in feature files. It is a plain-text language designed to be readable by both humans and machines.
12. What are Regular Expressions in Cucumber?
Regular expressions (regex) are used in step definitions to capture values from the feature file steps.
Example:
ruby
/^I have (\d+) apples$/
13. What is BDD (Behavior Driven Development)?
BDD is a software development approach that encourages collaboration between developers, testers, and business stakeholders.
It builds upon TDD (Test Driven Development), focusing on user behavior instead of just testing functions.
14. What software/tools are needed to run Cucumber web test cases?
To execute Cucumber web tests, you need:
- Ruby & DevKit
- Cucumber gem
- IDE (e.g., RubyMine or ActiveState)
- Watir (browser automation)
- RSpec, Ansicon (optional for output formatting)
15. What does the features/support folder contain?
The features/support folder contains initialization code and hooks that are executed before the test scenarios.
It’s ideal for:
- Environment setup
- Global configurations
16. What is a Feature File?
A Feature File contains:
- Feature: Brief description of the test functionality.
- Scenario: Each test case.
- Scenario Outline: Data-driven testing.
- Given, When, Then: Steps written in Gherkin syntax.
17. What is Selenium?
Selenium is a web automation tool used to automate browser-based applications.
It supports multiple languages including Java, Python, C#, and Ruby.
18. Why integrate Cucumber with Selenium?
Cucumber and Selenium are often used together to:
- Automate browser-based BDD tests
- Provide readable test cases
- Involve non-technical stakeholders
19. What are the core advantages of using Cucumber?
- Supports collaborative testing
- Easy to understand test cases
- Encourages code reusability
- Enables faster feedback cycles
20. What is a Step Definition?
A Step Definition connects feature file steps to executable code.
It executes test logic on the Application Under Test and compares the actual outcome with expected results.
👉Bonus Tip
These Cucumber interview questions are not only useful for job interviews but also for viva, oral exams, or automation testing certifications.