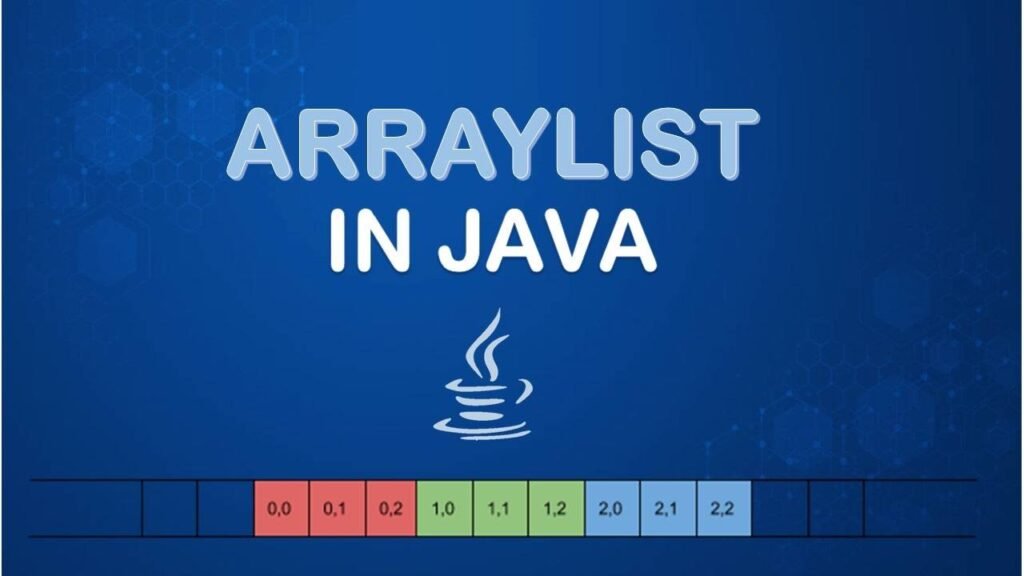
ArrayList in Java
➡️What is ArrayList in Java?
ArrayList in Java is a resizable array implementation of the List
interface in the Java Collections Framework. Unlike arrays, which have a fixed size, an ArrayList can dynamically grow or shrink based on the number of elements stored in it. This makes it a powerful data structure for handling dynamic data.
➡️Why Use ArrayList Instead of Arrays?
- Dynamic Sizing: Unlike arrays, which have a fixed size, an ArrayList automatically expands when new elements are added and shrinks when elements are removed.
- Efficient Retrieval: Provides fast element retrieval using indexing.
- Flexible Manipulation: Supports various operations like insertion, deletion, and searching.
- Easy Iteration: Can be easily traversed using loops and iterators.
➡️How to Create an ArrayList in Java?
To declare an ArrayList, use the following syntax:
import java.util.ArrayList;
ArrayList<Object> list = new ArrayList<Object>();
Here, Object
can be replaced with a specific data type such as String
, Integer
, or CustomClass
.
➡️Commonly Used Methods in ArrayList:
1. Adding Elements to an ArrayList:
Use the add()
method to insert elements into an ArrayList.
Syntax:
list.add(Object o);
Example:
ArrayList<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
System.out.println(names); // Output: [Alice, Bob, Charlie]
2. Removing Elements from an ArrayList:
Use the remove()
method to delete elements from an ArrayList.
Syntax:
list.remove(Object o); // Remove by value
list.remove(int index); // Remove by index
Example:
names.remove("Bob"); // Removes "Bob" from the list
names.remove(1); // Removes the second element
3. Getting the Size of an ArrayList:
The size()
method returns the number of elements in the list.
Syntax:
int size = list.size();
Example:
System.out.println("Size of ArrayList: " + names.size());
4. Checking If an Element Exists:
Use the contains()
method to check if an element is present in the list.
Syntax:
boolean exists = list.contains(Object o);
Example:
System.out.println(names.contains("Alice")); // Output: true
➡️Java ArrayList Example Program:
import java.util.ArrayList;
class TestArrayList {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
System.out.println("Size at start: " + list.size());
list.add("A");
list.add("B");
list.add("C");
System.out.println("Size after adding elements: " + list.size());
System.out.println("Elements: " + list);
list.remove("A");
System.out.println("After removing 'A': " + list);
list.remove(1);
System.out.println("After removing index 1: " + list);
System.out.println("Contains 'B'? " + list.contains("B"));
}
}
Output:
Size at start: 0
Size after adding elements: 3
Elements: [A, B, C]
After removing 'A': [B, C]
After removing index 1: [B]
Contains 'B'? true
➡️Advantages of Using ArrayList in Java:
- Dynamic Resizing: Adjusts automatically as elements are added or removed.
- Index-Base****d Access: Allows retrieval of elements using an index.
- Built-in Methods: Provides powerful methods for list operations.
- Memory Efficiency: Uses only the required memory for stored elements.
➡️Conclusion:-
ArrayList in Java is a flexible and efficient way to manage collections of dynamic data. Its ability to resize dynamically, coupled with built-in methods for adding, removing, and searching elements, makes it an essential data structure for Java developers. If you’re working with data that changes frequently, ArrayList is an excellent choice over traditional arrays.