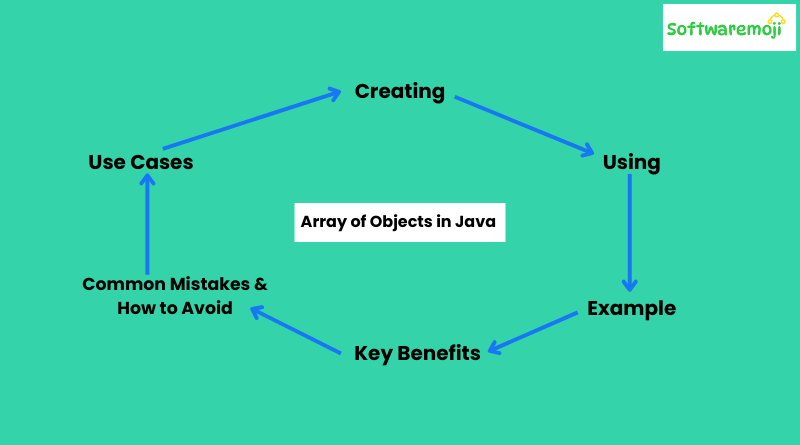
✅Array of Objects in Java:-
👉Declaring an Array of Objects in Java
You can declare an array of objects in Java using syntax like:ClassName[] arrayName = new ClassName[size];
This allows you to create an array that holds references to objects of the specified class.
Accessing Objects in an Array in Java
Objects in an array can be accessed using the index. For example:students[0] = new Student();
This assigns a new Student
object to the first element of the students
array.
Iteration Over Array of Objects
You can iterate through an array of objects using loops such as a for
loop:for (int i = 0; i < students.length; i++) { students[i].displayDetails(); }
Array of Objects vs. ArrayList in Java
While an array of objects has a fixed size, an ArrayList
in Java is dynamic, meaning its size can change as elements are added or removed. For dynamic collections, an ArrayList
is often preferred.
Common Pitfalls with Arrays of Objects
One common issue with arrays of objects is that if you don’t initialize the objects within the array, they may contain null
references, leading to NullPointerExceptions
during runtime.
✅What is an Array of Objects in Java?
In Java, an array of objects is a data structure that holds multiple objects of the same class. Unlike primitive arrays (e.g., int[]
, double[]
), an array of objects stores references to object instances. This approach allows handling multiple objects efficiently, making it a powerful feature in Java programming.
✅How to Create an Array of Objects in Java?
To create an array of objects in Java, follow these steps:
- Declare an array of object references.
- Instantiate the array with a specific size.
- Initialize each array element by creating an object.
Syntax:
javaClassName arrayName[] = new ClassName[array_size];
or
javaClassName[] arrayName = new ClassName[array_size];
✅Example Program: Creating and Using an Array of Objects:
javaclass Account {
int accountNumber;
double balance;
// Constructor
Account(int accNum, double bal) {
this.accountNumber = accNum;
this.balance = bal;
}
// Method to display account details
void display() {
System.out.println("Account Number: " + accountNumber);
System.out.println("Balance: $" + balance);
}
}
public class ObjectArrayExample {
public static void main(String[] args) {
// Step 1: Create an array of object references
Account accounts[] = new Account[3];
// Step 2: Initialize objects in the array
accounts[0] = new Account(101, 5000);
accounts[1] = new Account(102, 7000);
accounts[2] = new Account(103, 9000);
// Step 3: Display the details of each account
for (int i = 0; i < accounts.length; i++) {
System.out.println("Account " + (i + 1) + " details:");
accounts[i].display();
System.out.println();
}
}
}
Output:
yamlAccount 1 details:
Account Number: 101
Balance: $5000.0
Account 2 details:
Account Number: 102
Balance: $7000.0
Account 3 details:
Account Number: 103
Balance: $9000.0
✅Key Benefits of Using Arrays of Objects in Java:-
- Efficient Storage – Allows managing multiple objects in a single array.
- Easy Iteration – Enables processing objects using loops.
- Better Code Organization – Helps structure data effectively in applications.
- Memory Optimization – Stores references instead of actual objects, optimizing memory usage.
✅Use Cases of Arrays of Objects in Java:-
- Banking Systems (to store multiple customer accounts).
- Student Management Systems (to store student records).
- Employee Databases (to manage employee details).
- Inventory Systems (to store product details in e-commerce platforms).
✅Common Mistakes & How to Avoid Them:
- Not Instantiating Objects Before Use
- Mistake: javaCopyEdit
Account accounts[] = new Account[2]; accounts[0].display(); // Causes NullPointerException
- Solution: Initialize objects before calling methods.
- Mistake: javaCopyEdit
- Using Wrong Index
- Always check the array length before accessing elements to prevent
ArrayIndexOutOfBoundsException
.
- Always check the array length before accessing elements to prevent
- Forgetting to Allocate Memory
- Just declaring an array does not create objects. You must instantiate each element separately.
✅Better Search Ranking:-
- Java Array of Objects Example.
- How to create an array of objects in Java.
- Java Object Array with Examples.
- Arrays in Java with Objects.
- Java Object Array Explained.
- Java Array of Class Objects.
- Java Data Structures Array of Objects.
By understanding arrays of objects in Java, developers can write efficient, scalable, and structured code for real-world applications. 🚀.