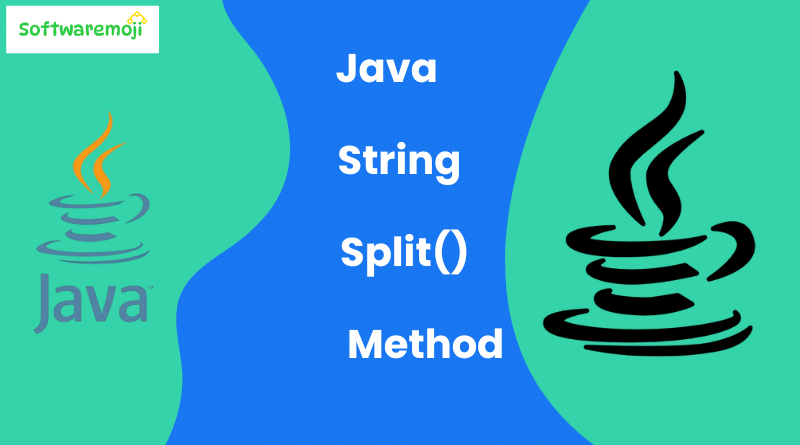
👉Java String split() Method:
➡️The Java split()
method is used to divide a string into an array of substrings based on a specified delimiter.
➡️It is a powerful tool for parsing data in Java, allowing you to break down complex strings into manageable parts.
➡️The split()
method in Java helps you easily manipulate strings, especially when dealing with CSV or space-separated data.
➡️By using regular expressions, the split()
method offers advanced pattern matching capabilities to split strings efficiently.
➡️When working with user inputs or file data, the split()
method is indispensable for extracting meaningful information.
➡️Java’s split()
method can be controlled with the limit parameter, allowing you to specify the maximum number of splits.
➡️With Java’s split()
method, you can separate string values into a clean, structured format for further processing or storage.
➡️It’s important to remember that the split()
method can return an empty string array if the delimiter doesn’t match anything in the string.
➡️The split()
method is part of Java’s String class and works seamlessly with regular expressions for more complex string manipulation.
➡️Understanding how to use the split()
method is essential for efficient string handling and data parsing in Java programming.
➡️The split()
method in Java is a powerful tool for breaking a string into multiple parts based on a specified delimiter. It is commonly used in text processing, data parsing, and handling user inputs.
👉What is the split() Method in Java?
The split()
method allows you to divide a string into an array of substrings based on a specific delimiter or pattern. This method is useful when working with structured data such as CSV files, logs, or any string that follows a specific format.
👉Syntax of split() Method:
javapublic String[] split(String regex)
public String[] split(String regex, int limit)
👉Parameters:
- regex: A regular expression (delimiter) used for splitting the string.
- limit: The maximum number of splits. If set to
0
or omitted, it returns all matching substrings.
👉Splitting a String in Java with a Delimiter:
👉Example 1: Splitting a String by Comma (,)
Let’s say you have a string containing names separated by commas, and you need to extract individual names.
👉Java Code:
javaclass SplitExample {
public static void main(String[] args) {
String str = "Apple, Banana, Mango, Orange, Pineapple";
String[] fruits = str.split(", ");
for (String fruit : fruits) {
System.out.println(fruit);
}
}
}
Output:
mathematicaApple
Banana
Mango
Orange
Pineapple
👉Using the split() Method with a Limit
Sometimes, you may want to limit the number of splits. This is useful when you need to retain part of the original string after a certain number of splits.
👉Java String split() Method Example 2: Limiting the Number of Splits–
javaclass SplitExampleLimit {
public static void main(String[] args) {
String str = "Java, Python, C++, JavaScript, PHP";
String[] languages = str.split(", ", 3);
for (String lang : languages) {
System.out.println(lang);
}
}
}
Output:
mathematicaJava
Python
C++, JavaScript, PHP
👉Java String split() Method Splitting a String in Java by Space:
If you need to break a sentence into individual words, you can split it using whitespace (\\s
).
👉Example 3: Splitting a String by Spaces–
javapublic class SplitBySpace {
public static void main(String[] args) {
String sentence = "Welcome to Java Programming";
String[] words = sentence.split("\\s");
for (String word : words) {
System.out.println(word);
}
}
}
Output:
cssWelcome
to
Java
Programming
👉Java String split() Method Benefits of String Manipulation in Java:
- Improved Data Processing: When handling web content, extracting and processing text efficiently using
split()
can improve website performance. - Parsing Logs & Reports: If you’re working with analytics or user logs, splitting text data is essential for structuring and analyzing information.
- Handling User Input: When processing forms or search queries, splitting user-provided data can help in better indexing and content customization.
👉Java String split() Method Final Thoughts:-
The Java split()
method is a must-know function for developers dealing with string manipulation. Whether you’re working on data extraction, text processing, or parsing user inputs, mastering this method will enhance your coding efficiency.