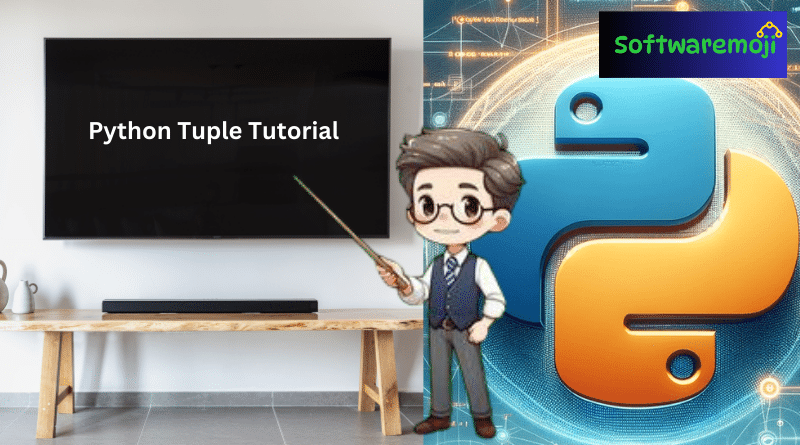
👉Tutorial-2: Python Dictionary Tutorial
👉Tutorial-3: Python Dictionary Append
👉Tutorial-4: Python Operators Explained
👉Tutorial-5: Python Not Equal Operator
👉Tutorial-6: Python Array Tutorial
👉Tutorial-7: Python 2D Arrays
👉Python Tuple Tutorial
👉Python Tuple – Comprehensive Guide with Examples
Python Tuple Tutorial : In Python, tuples are versatile data structures that store multiple elements. They are immutable, meaning their values cannot be changed after assignment. This guide explores key concepts such as tuple packing, unpacking, slicing, and comparison.
👉What is Tuple Matching in Python?
Python Tuple Tutorial : Tuple matching groups tuples by matching specific elements. Using dictionaries, we can effectively manage and group tuples based on matching values.
👉Python Tuple Syntax
Python Tuple Tutorial :
Here’s how you can create tuples in Python:
python
# Creating a tuple
Tup = (‘Jan’, ‘Feb’, ‘March’)
# Empty tuple
tup1 = ()
# Single-value tuple (comma is mandatory)
Tup1 = (50,)
👉Python Tuple Tutorial : Tuple Assignment
Python allows tuple assignment, which assigns multiple values at once.
👉Example:
python
tup1 = (‘Robert’, ‘Carlos’, ‘1965’, ‘Terminator’, ‘Actor’, ‘Florida’)
tup2 = (1, 2, 3, 4, 5, 6, 7)
print(tup1[0]) # Output: Robert
print(tup2[1:4]) # Output: (2, 3, 4)
👉Packing and Unpacking Tuples
- Packing: Assigning values into a tuple.
- Unpacking: Extracting values from a tuple into variables.
👉Example:
python
x = (“Software”, 20, “Education”) # Packing
(company, emp, profile) = x # Unpacking
print(company) # Output: Software
print(emp) # Output: 20
print(profile) # Output: Education
👉Comparing Tuples
Python’s comparison operators can compare tuples element by element.
👉Example:
python
# Case 1
a = (5, 6)
b = (1, 4)
if a > b:
print(“a is bigger”)
else:
print(“b is bigger”)
# Output: a is bigger
👉Using Tuples as Dictionary Keys
Since tuples are immutable and hashable, they can be used as dictionary keys.
👉Example:
python
directory = {(‘Smith’, ‘John’): ‘123-456-7890’}
for last, first in directory:
print(first, last, directory[last, first])
👉Tuple to Dictionary Conversion
Tuples can be converted into dictionary key-value pairs using .items().
👉Example:
python
a = {‘x’: 100, ‘y’: 200}
b = list(a.items())
print(b) # Output: [(‘x’, 100), (‘y’, 200)]
👉Deleting Tuples
Tuples are immutable, meaning individual elements cannot be deleted. However, you can delete the entire tuple using del.
👉Example:
python
tup = (‘a’, ‘b’, ‘c’)
del tup
👉Tuple Slicing
Slicing allows you to extract specific elements from a tuple.
👉Example:
python
x = (“a”, “b”, “c”, “d”, “e”)
print(x[2:4]) # Output: (‘c’, ‘d’)
👉Built-in Tuple Functions
Python offers several useful built-in functions for tuples:
- all()
- any()
- enumerate()
- max()
- min()
- sorted()
- len()
- tuple()
👉Advantages of Tuples Over Lists
✅ Faster iteration than lists
✅ Immutable elements provide data integrity
✅ Tuples can be used as keys in dictionaries
👉Summary
- Python tuples provide efficient data storage for immutable data.
- Tuple packing and unpacking enhance readability and flexibility.
- Tuples support element-wise comparison.
- Tuples can be keys in dictionaries for composite key requirements.
- Though immutable, entire tuples can be deleted using del.