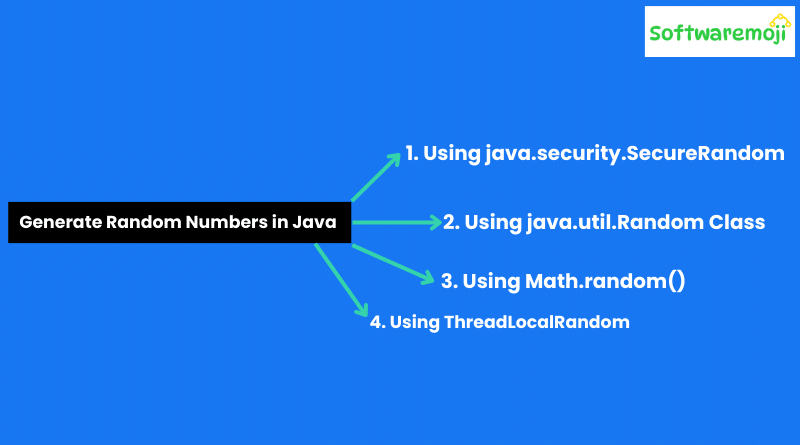
🔥How to Generate Random Numbers in Java:
“Learn how to generate random numbers in Java using simple and efficient methods.”
“Java provides multiple ways to generate random numbers, perfect for games, simulations, and security.”
“Discover the use of Random
, ThreadLocalRandom
, and SecureRandom
in Java for random number generation.”
“Master Java’s built-in tools to generate integers, floats, and even secure random values.”
“Generate random numbers in Java for all your programming needs—from basic to cryptographic purposes.”
🔥Introduction:-
Generating random numbers is a common requirement in Java applications, whether for simulations, cryptography, or simple lottery programs. Java provides multiple ways to generate random numbers, including the Random
class and the Math.random()
method.
Generating random numbers in Java is a common task in software development, especially for games, simulations, and testing. Java provides several ways to generate random numbers, the most commonly used being the java.util.Random
class and Math.random()
method.
In this guide, we will explore the best ways to generate random numbers efficiently in Java.
🔥Methods to Generate Random Numbers in Java:
Java provides two primary methods to generate random numbers:
- Using
java.util.Random
class – Suitable for generating random integers, floating points, and boolean values. - Using
Math.random()
– A simple way to generate random double values between0.0
and1.0
. - Using
java.security.SecureRandom
– A cryptographically secure method for generating random numbers. - Using
ThreadLocalRandom
– A more efficient way to generate random numbers in multi-threaded applications.
1. Using java.util.Random
Class:
The Random
class from java.util
package provides methods like nextInt()
, nextDouble()
, and nextLong()
to generate random numbers.
🔥Example: Generate 10 Random Integers Between 0 and 100:
javaimport java.util.Random;
public class RandomNumbers {
public static void main(String[] args) {
Random random = new Random();
for (int i = 0; i < 10; i++) {
int randomNumber = random.nextInt(100); // Generates number between 0 and 99
System.out.println("Random Number: " + randomNumber);
}
}
}
Output Example:
mathematicaRandom Number: 45
Random Number: 78
Random Number: 21
Random Number: 92
Random Number: 37
Random Number: 14
...
🔹 The nextInt(100)
method ensures the random number is between 0
(inclusive) and 100
(exclusive).
2. Using Math.random()
The Math.random()
method generates a random double value between 0.0
and 1.0
.
🔥Example: Generate 10 Random Double Numbers:
javapublic class MathRandomExample {
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
double randomValue = Math.random(); // Generates a number between 0.0 and 1.0
System.out.println("Random Double: " + randomValue);
}
}
}
🔹 To get an integer range, you can use:
javaint randomInt = (int)(Math.random() * 100); // Generates between 0 and 99
3. Using java.security.SecureRandom
(For Security):
If you need a cryptographically secure random number, use SecureRandom
.
🔥Example: Generate Secure Random Number:
javaimport java.security.SecureRandom;
public class SecureRandomExample {
public static void main(String[] args) {
SecureRandom secureRandom = new SecureRandom();
int secureNum = secureRandom.nextInt(100); // Generates between 0 and 99
System.out.println("Secure Random Number: " + secureNum);
}
}
🔹 Ideal for password generation and security-related tasks.
4. Using ThreadLocalRandom
(For High Performance):
For better performance in multi-threaded applications, ThreadLocalRandom
is recommended.
🔥How to Generate Random Numbers in Java Example: Generate Random Numbers Using ThreadLocalRandom
–
javaimport java.util.concurrent.ThreadLocalRandom;
public class ThreadLocalRandomExample {
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
int randomNum = ThreadLocalRandom.current().nextInt(1, 101); // Generates 1 to 100
System.out.println("ThreadLocalRandom Number: " + randomNum);
}
}
}
🔹 Faster than Random
in multi-threaded applications.
🔥How to Generate Random Numbers in Java Comparison of Methods:
Method | Best Use Case | Range Customization | Performance |
---|---|---|---|
Random | General purpose random numbers | Yes | Good |
Math.random() | Simple random doubles | No (fixed 0.0 – 1.0) | Fast |
SecureRandom | Cryptographic security | Yes | Slower but secure |
ThreadLocalRandom | Multi-threaded apps | Yes | Best performance |
🔥How to Generate Random Numbers in Java Conclusion:-
Java provides multiple ways to generate random numbers, each with its own use case.
- Use
Random
for general-purpose random numbers. - Use
Math.random()
for simple floating-point numbers. - Use
SecureRandom
for security-sensitive applications. - Use
ThreadLocalRandom
for high-performance applications.
By choosing the right method, you can optimize your Java application for speed, security, and efficiency. 🚀