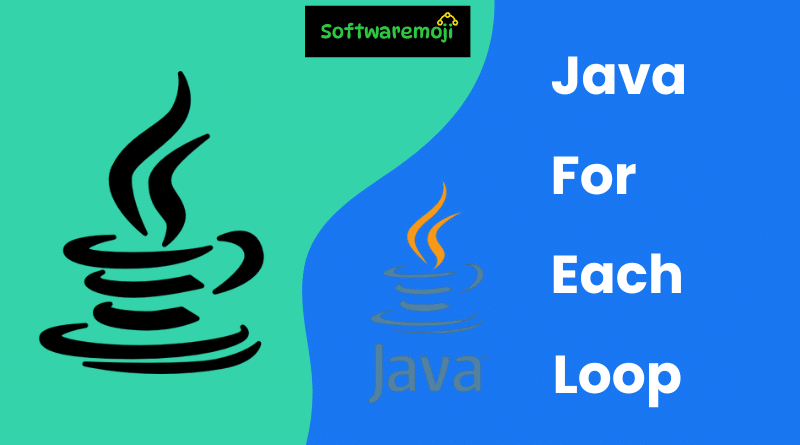
➡️Java For-Each Loop:
- The Java for-each loop simplifies iteration over arrays and collections with clean syntax.
- For-each loop in Java enhances code readability and reduces the chance of errors.
- Java’s for-each loop is ideal for accessing each element without using an index.
- Use for-each in Java to avoid the boilerplate code of traditional for loops.
- Java for-each loop supports iteration over Lists, Sets, Arrays, and other Iterable types.
➡️Introduction to For-Each Loop in Java:
👉Tutorial-1:-Java Switch Statement.
The for-each loop in Java is an enhanced version of the traditional for
loop that is specifically used for traversing arrays and collections. It simplifies iteration by eliminating the need for an explicit index variable, making the code more readable and efficient.
➡️Why Use For-Each Loop?
✅ Simpler Syntax – Reduces boilerplate code.
✅ Avoids Indexing Errors – No need to manually manage loop counters.
✅ Enhances Readability – The code is clean and easy to understand.
✅ Works for Collections – Can be used with Lists, Sets, and Maps apart from arrays.
⭐ 1. Simplifies Array and Collection Iteration:
The Java for-each loop provides a cleaner and more readable way to iterate through arrays and collections, eliminating the need for index management.
⭐ 2. Enhances Code Readability and Maintenance:
With its concise syntax, the for-each loop reduces boilerplate code, making Java programs easier to understand and maintain, especially for beginners.
⭐ 3. Prevents Index-Out-of-Bounds Errors:
Since there is no use of explicit indexing, the for-each loop automatically prevents common errors like ArrayIndexOutOfBoundsException
.
➡️Syntax of For-Each Loop:
javafor (DataType var : arrayOrCollection) {
// Code to execute for each element
}
DataType
→ The type of elements in the array or collection.var
→ A temporary variable that holds each element.arrayOrCollection
→ The array or collection to be iterated.
➡️For-Each Loop Example in Java (Array Traversal):
Let’s consider a String array and iterate through its elements using both a traditional for loop and a for-each loop.
Example Code:
javaclass ForEachExample {
public static void main(String[] args) {
String[] languages = {"Java", "Python", "C++", "JavaScript"};
// Using traditional for loop
System.out.println("Using Traditional For Loop:");
for (int i = 0; i < languages.length; i++) {
System.out.println(languages[i]);
}
// Using for-each loop
System.out.println("\nUsing For-Each Loop:");
for (String lang : languages) {
System.out.println(lang);
}
}
}
Expected Output:
mathematicaUsing Traditional For Loop:
Java
Python
C++
JavaScript
Using For-Each Loop:
Java
Python
C++
JavaScript
➡️For-Each Loop for Collections (ArrayList Example):
- With for-each, Java developers write fewer lines of code and achieve better clarity.
- The for-each loop in Java is a safer alternative when modification of the collection is not required.
- Java for-each loop internally uses an Iterator, but offers a more concise way to loop.
- Boost Java performance and maintainability by using for-each where applicable.
- In Java 8 and beyond, for-each can be combined with lambda expressions for modern coding practices.
Apart from arrays, for-each loops are widely used with ArrayLists, which are part of Java’s Collection Framework.
javaimport java.util.ArrayList;
class ForEachWithArrayList {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(10);
numbers.add(20);
numbers.add(30);
numbers.add(40);
System.out.println("Iterating through ArrayList using For-Each Loop:");
for (int num : numbers) {
System.out.println(num);
}
}
}
Expected Output:
vbnetIterating through ArrayList using For-Each Loop:
10
20
30
40
➡️Limitations of For-Each Loop:
❌ Cannot Modify List Items – It doesn’t allow modifying elements while iterating.
❌ No Reverse Traversal – Unlike traditional loops, it doesn’t support iterating backward.
❌ Cannot Access Index – If you need an index reference, use a normal for loop.
➡️Conclusion:
The for-each loop in Java simplifies iteration, reduces code complexity, and improves readability. It is best suited for scenarios where elements are only being accessed and not modified during iteration. However, if you need index-based access or modifications, using a traditional for loop is recommended.
Would you like a more detailed example with Maps or Sets? 🚀