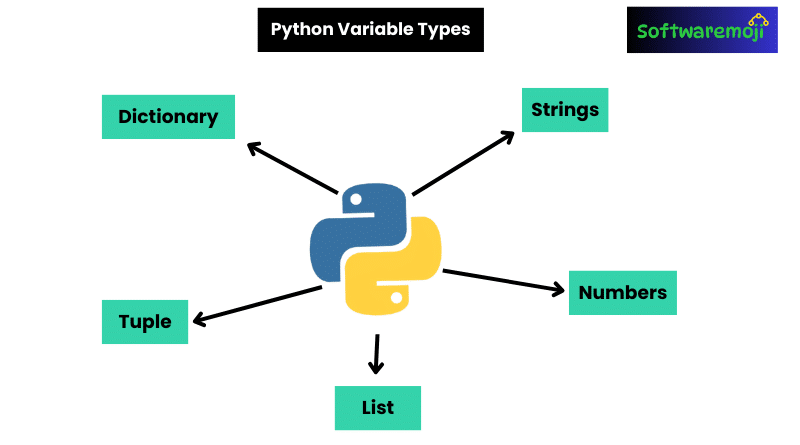
👉Python Variables : How to Declare, Use, and Manage Variable Types
👉What is a Variable in Python?
Python Variables : A Python variable is a reserved memory location that stores values. It acts as a reference point for data that the computer can process.
👉Python Variable Types
Python Variables : Python supports various data types like:
- Numbers
- List
- Tuple
- Strings
- Dictionary
Python variables can be declared using any name or alphabet combinations such as a, abc, x1, etc.
👉How to Declare and Use a Variable in Python
How to Declare and Use a Variable in Python
👉Example 1: Declaring a Variable
# Declare a variable
a = 100
print(a)
Output:
100
👉Re-declare a Variable
Python Variables : Python allows you to re-declare variables even after assigning them a value.
👉Example 2: Re-declaring a Variable
f = 0
print(f) # Output: 0
f = ‘moji’
print(f) # Output: moji
👉Python String Concatenation and Variables
Python Variables: Python requires explicit conversion of numbers to strings when concatenating with text.
👉Example 3: String Concatenation
a = “Software”
b =Moji
print(a + str(b)) # Output: moji
👉Python Variable Types: Local vs Global
Python variables can be either local or global.
👉Example 4: Global and Local Variables
# Global variable
global_var = 101
print(global_var) # Output: 101
def someFunction():
# Local variable
local_var = ‘I am learning Python’
print(local_var) # Output: I am learning Python
someFunction()
print(global_var) # Output: 101
👉Example 5: Changing Global Variables
f = 101
print(f) # Output: 101
def someFunction():
global f
print(f) # Output: 101
f = “Changing global variable”
someFunction()
print(f) # Output: Changing global variable
👉How to Delete a Variable in Python
You can delete a variable using the del command.
👉Example 6: Deleting a Variable
f = 11
print(f) # Output: 11
del f
print(f) # Error: variable not defined
👉Summary
- Variables in Python store data and are declared with simple names.
- Python allows variables to be re-declared.
- String concatenation requires explicit conversion of numbers to strings.
- Python supports local and global variables, with the global keyword enabling changes to global variables inside functions.
- Variables can be deleted using the del keyword.