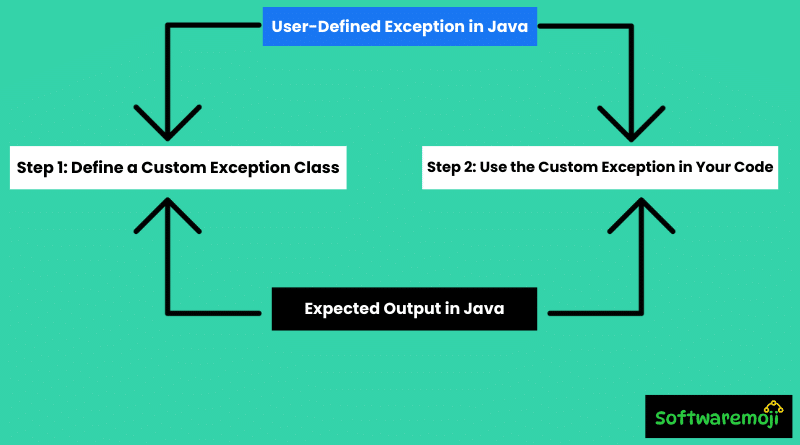
📌User-Defined Exception in Java:
User-defined exceptions in Java allow developers to create custom error-handling mechanisms tailored to specific application needs.
By using custom exceptions in Java, developers can improve code readability and enforce business rules effectively.
A Java user-defined exception is created by extending the Exception
or RuntimeException
class, providing flexibility in error handling.
With custom Java exceptions, it’s easier to debug and track issues specific to your application domain.
User-defined exceptions in Java programming enhance modularity by isolating specific error conditions into separate classes.
Creating your own exception class in Java helps to handle application-specific errors more efficiently.
📌User-Defined Exception in Java Introduction:
Java provides a powerful exception handling mechanism to deal with runtime errors. While Java offers many built-in exceptions, sometimes they are not sufficient to handle specific application-level errors. In such cases, user-defined exceptions in Java (also known as custom exceptions) are used. This article explains what a user-defined exception is, how to create one, and why it’s important in real-time applications.
📌What is a User-Defined Exception in Java?
In Java, a user-defined exception (also known as a custom exception) is a special type of exception that developers create to handle specific error conditions that are not covered by built-in exceptions. These exceptions are created by extending the Exception
class and using the throw
keyword to trigger them when needed.
📌Why Use User-Defined Exceptions?
Creating custom exceptions provides several benefits, including:
- Handling Specific Application Errors: Standard exceptions like
NullPointerException
orArrayIndexOutOfBoundsException
may not always cover the errors in your application. User-defined exceptions help address specific issues. - Improving Code Readability: By defining meaningful exception names, developers can easily understand and debug errors.
- Enhancing Code Maintainability: With custom exceptions, you can centralize error-handling logic, making future updates easier.
- Following Best Practices: Custom exceptions help maintain clean and structured error-handling mechanisms in Java applications.
📌How to Create a User-Defined Exception in Java:
Using user-defined exception handling in Java improves software robustness and reduces dependency on generic error messages.
📌Step 1: Define a Custom Exception Class:
To create a user-defined exception, extend the Exception
class and override the toString()
method for a meaningful error message.
java// Custom Exception Class
class InvalidAgeException extends Exception {
private int age;
// Constructor
public InvalidAgeException(int age) {
this.age = age;
}
// Custom error message
public String toString() {
return "InvalidAgeException: Age " + age + " is not valid.";
}
}
📌Step 2: Use the Custom Exception in Your Code:
Now, use the custom exception in a program and handle it using try-catch
.
javapublic class CustomExceptionDemo {
static void checkAge(int age) throws InvalidAgeException {
if (age < 18) {
throw new InvalidAgeException(age); // Throw custom exception
} else {
System.out.println("Access granted. Age: " + age);
}
}
public static void main(String[] args) {
try {
checkAge(16); // This will trigger the custom exception
} catch (InvalidAgeException e) {
System.out.println(e);
}
}
}
Expected Output:
vbnetInvalidAgeException: Age 16 is not valid.
📌User-Defined Exception in Java Best Practices for Using User-Defined Exceptions:
- Use Meaningful Names: Name your exceptions based on the error scenario (e.g.,
InvalidTransactionException
). - Extend the Right Class: Use
Exception
for checked exceptions andRuntimeException
for unchecked exceptions. - Provide Constructors: Allow custom messages and error codes for better debugging.
- Log Exceptions: Always log exceptions to identify issues efficiently.
- Avoid Overuse: Use custom exceptions only when necessary to prevent unnecessary complexity.
📌Benefits of User-Defined Exceptions:
- Improve code readability and clarity.
- Make debugging and logging easier.
- Provide custom error messages.
- Help in maintaining business logic separately.
📌Conclusion:
User-defined exceptions in Java allow developers to create meaningful error messages, improve code readability, and enhance maintainability. By defining and handling custom exceptions properly, Java applications become more robust and user-friendly.
User-defined exceptions in Java are a great way to make your code more robust and meaningful. By creating custom exceptions, developers can handle specific error scenarios with more control and clarity. It is a good practice to use them in large-scale applications where custom error management is required.