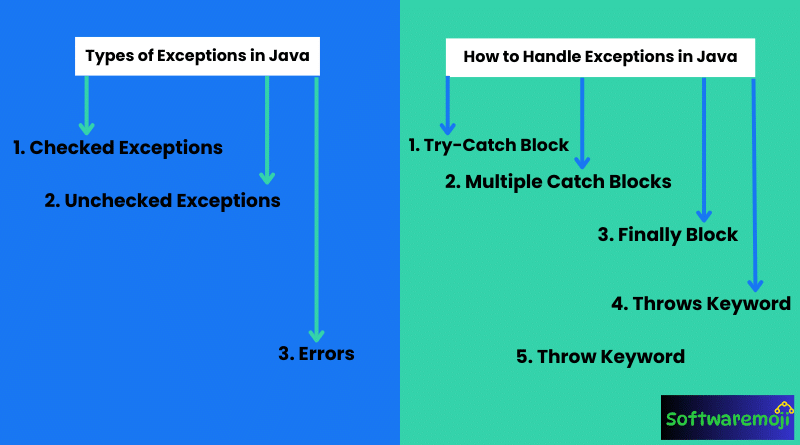
✅Exception Handling in Java:
Exception Handling in Java is a powerful mechanism that ensures smooth program execution even when unexpected errors occur.
Java uses try-catch blocks to handle exceptions, promoting robust and fault-tolerant applications.
✅What is an Exception in Java?
👉Tutorial-1:-User-Defined Exception in Java.
👉Tutorial-2:-Java Throws Keyword.
An exception in Java is an event that disrupts the normal flow of a program during execution. It is an object that contains information about an error, which is then passed to the runtime system. Exception handling is a crucial part of Java programming, ensuring robust applications by managing unexpected conditions like divide-by-zero errors, null references, or invalid input data.
✅Why is Exception Handling Important?
- Prevents program crashes due to unexpected errors
- Ensures smooth user experience
- Helps in debugging by providing meaningful error messages
- Improves maintainability and code readability
✅Types of Exceptions in Java:-
1. Checked Exceptions:
Checked exceptions are errors detected at compile time. These exceptions must be handled using a try-catch
block or declared using throws
.
Example: IOException
, SQLException
javaimport java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class CheckedExceptionExample {
public static void main(String[] args) {
try {
File file = new File("test.txt");
FileReader fr = new FileReader(file); // May throw FileNotFoundException
} catch (IOException e) {
System.out.println("File not found: " + e.getMessage());
}
}
}
2. Unchecked Exceptions (Runtime Exceptions):
Unchecked exceptions occur at runtime and do not require explicit handling. These are caused by logical programming errors.
Example: NullPointerException
, ArrayIndexOutOfBoundsException
, ArithmeticException
javapublic class UncheckedExceptionExample {
public static void main(String[] args) {
int num = 10, denom = 0;
int result = num / denom; // Throws ArithmeticException
System.out.println(result);
}
}
3. Errors:
Errors are serious system-level problems that should not be caught or handled in the program.
Example: StackOverflowError
, OutOfMemoryError
javapublic class ErrorExample {
public static void recursiveMethod() {
recursiveMethod(); // Causes StackOverflowError
}
public static void main(String[] args) {
recursiveMethod();
}
}
✅How to Handle Exceptions in Java:
1. Try-Catch Block:
The try
block contains code that may throw an exception, while the catch
block handles it.
javapublic class TryCatchExample {
public static void main(String[] args) {
try {
int[] arr = new int[5];
arr[10] = 100; // Throws ArrayIndexOutOfBoundsException
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
2. Exception Handling in Java Multiple Catch Blocks:
A program can have multiple catch
blocks for different exceptions.
javapublic class MultipleCatchExample {
public static void main(String[] args) {
try {
int a = 10 / 0; // Throws ArithmeticException
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero.");
} catch (Exception e) {
System.out.println("General exception occurred.");
}
}
}
3. Finally Block:
The finally
block always executes, regardless of whether an exception occurs.
javapublic class FinallyExample {
public static void main(String[] args) {
try {
int num = 10 / 0;
} catch (ArithmeticException e) {
System.out.println("Exception: " + e.getMessage());
} finally {
System.out.println("Finally block executed.");
}
}
}
4. Throws Keyword:
If a method does not handle an exception, it can declare it using throws
.
javaimport java.io.IOException;
public class ThrowsExample {
public static void riskyMethod() throws IOException {
throw new IOException("IO Error occurred");
}
public static void main(String[] args) {
try {
riskyMethod();
} catch (IOException e) {
System.out.println(e.getMessage());
}
}
}
5. Throw Keyword:
The throw
keyword is used to explicitly throw an exception.
javapublic class ThrowExample {
public static void validateAge(int age) {
if (age < 18) {
throw new IllegalArgumentException("Age must be 18 or above.");
}
}
public static void main(String[] args) {
validateAge(16);
}
}
✅Exception Handling in Java Best Practices for Exception Handling:
- Use Specific Exception Types – Catch specific exceptions rather than using
Exception
to improve debugging. - Use Finally Block for Resource Cleanup – Always close resources (files, database connections) in the
finally
block. - Avoid Empty Catch Blocks – Always log or print error messages.
- Use Custom Exceptions for Business Logic – Create user-defined exceptions for better clarity.
- Don’t Overuse Checked Exceptions – Use checked exceptions for recoverable errors and runtime exceptions for programming mistakes.
✅Exception Handling in Java Conclusion:-
Exception handling is a fundamental concept in Java that enhances application stability and robustness. By using try-catch-finally
, throws
, and throw
, developers can gracefully manage errors and provide a better user experience.
Would you like more advanced topics like custom exceptions, logging strategies, or best practices in production environments? 🚀