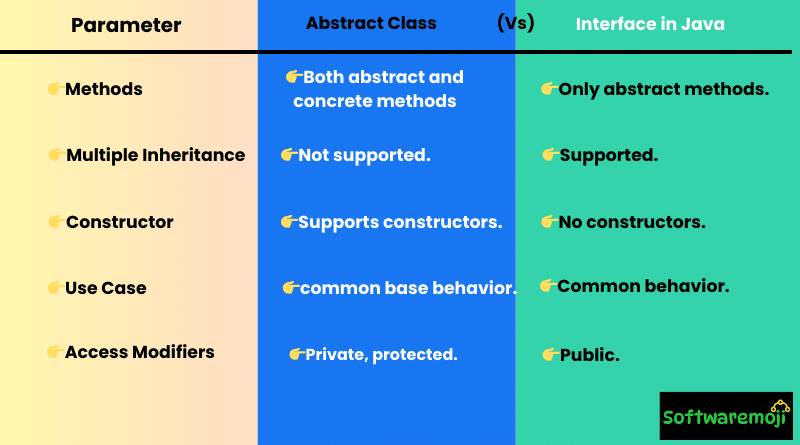
✅Abstract Class vs Interface in Java:
- Abstract classes in Java allow both abstract and concrete methods, providing a flexible blueprint for subclasses.
- Java interfaces support multiple inheritance, making them ideal for defining capabilities across unrelated classes.
- Use abstract classes when you want to share common code among closely related classes.
- Prefer interfaces in Java when you want to define a contract without enforcing any implementation.
- Abstract classes can have constructors and state (fields), which interfaces couldn’t have before Java 8.
- From Java 8 onwards, interfaces can have default and static methods, offering limited behavior sharing.
- Interfaces are better for defining APIs, while abstract classes are best for code reusability in inheritance hierarchies.
When working with Java, understanding the differences between abstract classes and interfaces is crucial for writing efficient, maintainable, and scalable code. Both are used for abstraction, but they serve different purposes and have distinct functionalities.
✅What is an Abstract Class?
An abstract class in Java is a class that cannot be instantiated and is meant to be extended by other classes. It can contain both abstract methods (without implementation) and concrete methods (with implementation).
✅Key Features of Abstract Classes:-
- Can have both abstract and non-abstract methods.
- Supports constructors and destructors.
- Can define fields (instance variables) and constants.
- Allows the use of access modifiers (private, protected, public).
- Provides a default implementation for methods, reducing code duplication.
- Supports single inheritance (a class can extend only one abstract class).
✅ When to Use an Abstract Class?
- When classes share a common base behavior.
- If you need to provide default method implementations.
- When you require access modifiers (like private, protected) for better encapsulation.
✅What is an Interface?
An interface in Java is a collection of abstract methods that define the behavior a class must implement. Unlike abstract classes, interfaces cannot have instance variables but can have public static final constants.
✅Key Features of Interfaces:-
- All methods are implicitly public and abstract.
- Cannot have constructors or destructors.
- No instance variables—only public static final constants.
- Supports multiple inheritance (a class can implement multiple interfaces).
- Helps achieve loose coupling between components.
✅ Abstract Class vs Interface in Java When to Use an Interface?
- When you need multiple inheritance in Java.
- If different classes share common behavior but are not related hierarchically.
- For designing plug-and-play architectures in large-scale applications.
- When defining functionalities rather than a hierarchy.
✅Abstract Class vs Interface: A Quick Comparison:-
Parameter | Abstract Class | Interface |
---|---|---|
Multiple Inheritance | Not supported | Supported |
Methods | Can have both abstract and concrete methods | Only abstract methods (until Java 8, which introduced default methods) |
Instance Variables | Yes, allows instance variables | No instance variables, only constants |
Access Modifiers | Can have private, protected, or public members | All members are public by default |
Constructor | Supports constructors | No constructors |
Use Case | Used for common base behavior among related classes | Used for defining common behavior for unrelated classes |
✅Abstract Class vs Interface in Java Best Practices for Using Abstract Classes and Interfaces:-
✅ Use an interface when you expect that multiple unrelated classes will implement it.
✅ Use an abstract class when you want to provide a common base class with default behavior.
✅ Prefer interfaces for defining capabilities, such as Runnable
, Comparable
, or Serializable
.
✅ Leverage Java 8+ features like default methods in interfaces when backward compatibility is needed.
✅Abstract Class vs Interface in Java Java Code Examples:-
Example of an Interface in Java-
javainterface Animal {
void makeSound(); // Abstract method
}
class Dog implements Animal {
public void makeSound() {
System.out.println("Dog barks!");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.makeSound();
}
}
Example of an Abstract Class in Java-
javaabstract class Vehicle {
abstract void startEngine(); // Abstract method
void stopEngine() { // Concrete method
System.out.println("Engine stopped.");
}
}
class Car extends Vehicle {
void startEngine() {
System.out.println("Car engine started.");
}
}
public class Main {
public static void main(String[] args) {
Vehicle myCar = new Car();
myCar.startEngine();
myCar.stopEngine();
}
}
✅Conclusion:-
Both abstract classes and interfaces are powerful tools in Java object-oriented programming. Choosing between them depends on the use case:
- If you need default method implementations, use an abstract class.
- If you need multiple inheritance and loose coupling, use an interface.
By understanding their differences and applying best practices, you can write scalable, maintainable, and efficient Java applications. 🚀.