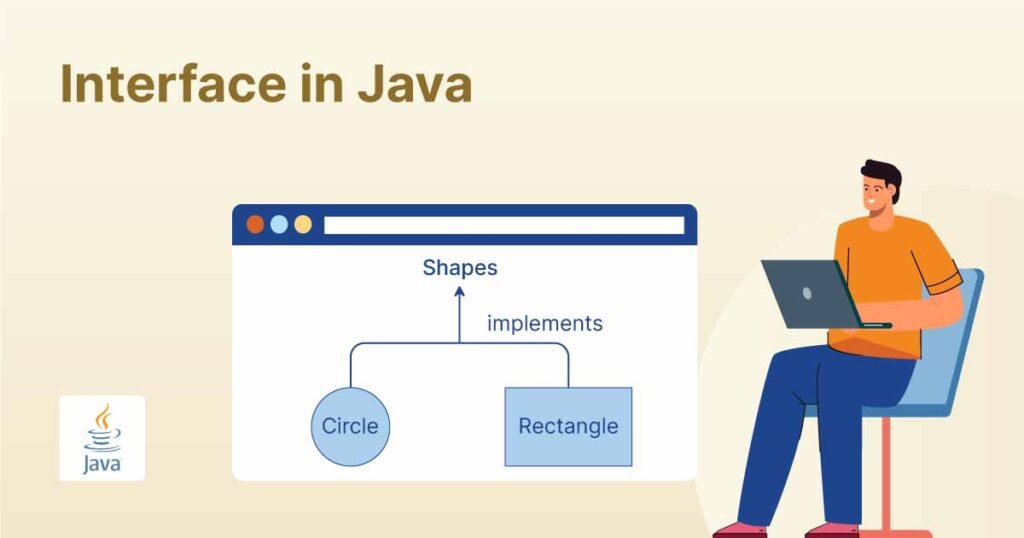
Java Interface
๐What is an Interface in Java?
An interface in Java is a blueprint for a class that contains abstract methods and static constants. It defines a contract that any implementing class must follow. Unlike classes, interfaces do not contain method implementationsโonly method signatures.
๐Key Features of Java Interfaces:-
โ 100% abstract (before Java 8)
โ Can contain default and static methods (Java 8 onwards)
โ Supports multiple inheritance
โ Cannot have instance variables.
โ Helps achieve loose coupling
๐Syntax of an Interface in Java:-
javainterface Animal {
void makeSound(); // Abstract method
}
A class can implement an interface using the implements
keyword:
javaclass Dog implements Animal {
public void makeSound() {
System.out.println("Dog barks");
}
}
๐Example: Implementing an Interface in Java:-
Let’s look at a practical example to understand how interfaces work in Java:
java// Defining an interface
interface Pet {
void play();
}
// Implementing the interface
class Dog implements Pet {
public void play() {
System.out.println("Dog is playing");
}
public static void main(String[] args) {
Pet myPet = new Dog();
myPet.play();
}
}
Output:
csharpDog is playing
๐Multiple Inheritance Using Interfaces:-
Java does not support multiple inheritance with classes but allows it with interfaces:
javainterface Animal {
void eat();
}
interface Bird {
void fly();
}
// A class implementing multiple interfaces
class Sparrow implements Animal, Bird {
public void eat() {
System.out.println("Sparrow eats grains");
}
public void fly() {
System.out.println("Sparrow is flying");
}
}
๐Interface vs Abstract Class: When to Use What?
Feature | Interface | Abstract Class |
---|---|---|
Method Implementation | Cannot have method implementations (before Java 8) | Can have both abstract and concrete methods |
Constructor | Not allowed | Allowed |
Inheritance | A class can implement multiple interfaces | A class can extend only one abstract class |
Purpose | Defines behavior (what a class should do) | Defines a base class (a common blueprint) |
โ
Use an Interface when multiple classes share behavior but are unrelated.
โ
Use an Abstract Class when classes share common functionality.
๐Must-Know Facts About Interfaces in Java:-
โ A class must implement all methods of an interface.
โ Interfaces support default and static methods (Java 8+).
โ An interface can extend multiple other interfaces.
โ Interface variables are public
, static
, and final
by default.
โ An interface cannot implement another interface (it extends instead).
๐Conclusion:-
Interfaces in Java play a crucial role in achieving abstraction, loose coupling, and multiple inheritance. They help design scalable and maintainable applications. By understanding when and how to use them, developers can write more efficient and modular code.
Would you like to explore more advanced topics like functional interfaces, default methods, and real-world examples? ๐.