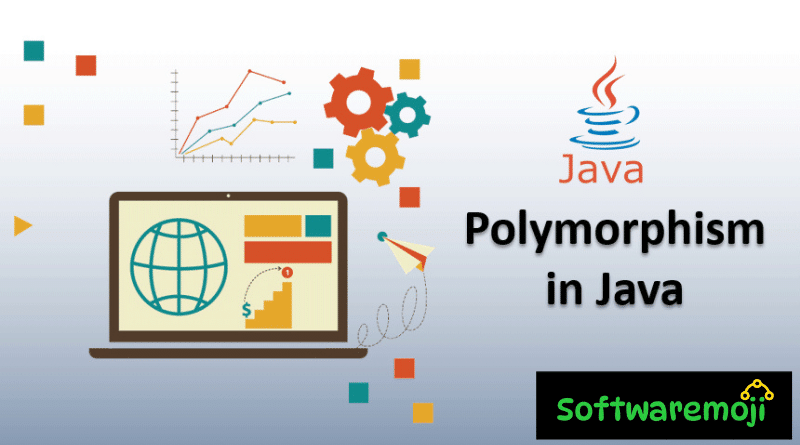
Polymorphism in Java: Static and Dynamic
➡️What is Polymorphism in Java?
Polymorphism in Java is an Object-Oriented Programming (OOP) concept that allows objects to take multiple forms. It enables a single interface to be used for different data types, making code more flexible and reusable. Java achieves polymorphism primarily through method overloading (static polymorphism) and method overriding (dynamic polymorphism).
➡️Types of Polymorphism in Java:-
1. Static Polymorphism (Compile-Time Polymorphism):-
Static polymorphism in Java is achieved through method overloading, where multiple methods in the same class share the same name but have different parameter lists. The method to be executed is determined at compile time.
Example of Method Overloading:
class MathOperations {
int sum(int a, int b) {
return a + b;
}
int sum(int a, int b, int c) {
return a + b + c;
}
double sum(double a, double b) {
return a + b;
}
}
public class Test {
public static void main(String[] args) {
MathOperations obj = new MathOperations();
System.out.println(obj.sum(10, 20));
System.out.println(obj.sum(10, 20, 30));
System.out.println(obj.sum(5.5, 2.5));
}
}
➡️Key Characteristics of Static Polymorphism:
- Method resolution happens at compile time.
- Achieved using method overloading.
- Faster execution as method binding happens early.
2. Dynamic Polymorphism (Run-Time Polymorphism):-
Dynamic polymorphism is achieved through method overriding, where a subclass provides a specific implementation of a method already defined in its superclass. The method that gets executed is determined at runtime based on the object type.
Example of Method Overriding:
class Animal {
void makeSound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void makeSound() {
System.out.println("Dog barks");
}
}
class Cat extends Animal {
void makeSound() {
System.out.println("Cat meows");
}
}
public class Test {
public static void main(String[] args) {
Animal myAnimal = new Dog(); // Reference of Animal, object of Dog
myAnimal.makeSound(); // Output: Dog barks
}
}
➡️Key Characteristics of Dynamic Polymorphism:
- Method resolution happens at runtime.
- Achieved using method overriding.
- Provides flexibility and allows implementing runtime-specific behavior.
➡️Difference Between Static and Dynamic Polymorphism:-
Feature | Static Polymorphism | Dynamic Polymorphism |
---|---|---|
Achieved By | Method Overloading | Method Overriding |
Method Binding | Compile-time | Runtime |
Execution Speed | Faster | Slower due to runtime method resolution |
Flexibility | Less flexible | More flexible |
Example | sum(int a, int b) , sum(double a, double b) | Overriding makeSound() in different classes |
➡️Super Keyword in Java:-
The super
keyword in Java is used to refer to the parent class methods and constructors from a subclass.
Example of Using super
Keyword:
class Parent {
void show() {
System.out.println("This is the parent class");
}
}
class Child extends Parent {
void show() {
super.show(); // Calls the parent class method
System.out.println("This is the child class");
}
}
public class Test {
public static void main(String[] args) {
Child obj = new Child();
obj.show();
}
}
Output:
This is the parent class
This is the child class
➡️Advantages of Polymorphism in Java:-
- Code Reusability – Reusing methods in different classes reduces redundancy.
- Flexibility – Enables dynamic method execution based on object type.
- Maintainability – Reduces modification effort by allowing changes in a single location.
- Extensibility – Allows adding new functionality without affecting existing code.
➡️Conclusion:-
Polymorphism in Java enhances the efficiency of Object-Oriented Programming by allowing multiple implementations under a unified interface. Static polymorphism (method overloading) resolves methods at compile time, whereas dynamic polymorphism (method overriding) determines the method execution at runtime. Understanding and implementing polymorphism improves code structure, flexibility, and maintainability in Java applications.