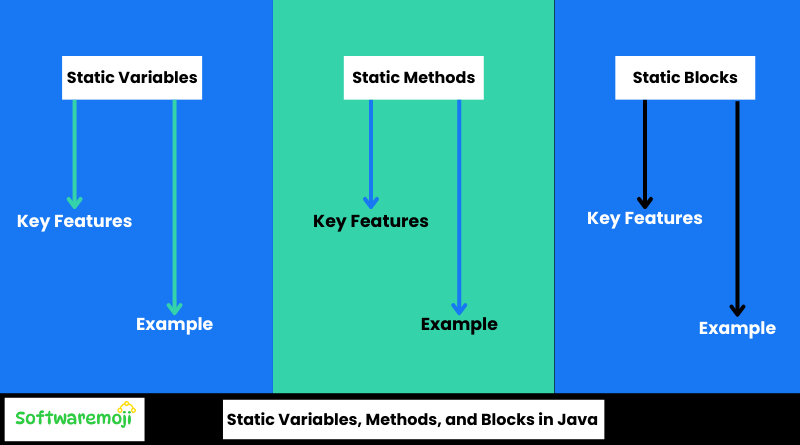
🔥 Static Variables:-
Static variables in Java are shared across all instances of a class, making memory management more efficient. Static methods in Java can be called without creating an object, which enhances performance and utility. Static blocks in Java are used to initialize static variables and are executed only once when the class is loaded. Static methods cannot access non-static data members directly, which encourages better program structure.
👉Tutorial-1:–Java Memory Management
🔥 What is a Static Variable in Java?
A static variable in Java is a class-level variable that is shared among all instances of the class. Unlike instance variables, which are created each time a new object is instantiated, static variables are initialized only once, when the class is loaded into memory.
Static blocks enhance the flexibility of Java by allowing pre-processing tasks during class loading.
Java’s static keyword supports object-oriented principles by allowing class-level operations without object instantiation.
🔥Key Features of Static Variables:
Static Variables, Methods, and Blocks in Java
- Belongs to the class rather than any specific object.
- Initialized only once at the start of execution.
- Shared by all instances of the class.
- Can be accessed directly using the class name (
ClassName.variableName
).
Syntax:
javaclass ClassName {
static int variableName;
}
Example Usage:
javaclass Student {
static int count = 0; // Static variable
Student() {
count++; // Increment count when a new object is created
}
void showCount() {
System.out.println("Number of students: " + count);
}
}
public class Demo {
public static void main(String[] args) {
Student s1 = new Student();
Student s2 = new Student();
Student s3 = new Student();
s3.showCount(); // Output: Number of students: 3
}
}
🔥What is a Static Method in Java?
A static method in Java is a method that belongs to the class rather than an instance of the class. It can only access static data and cannot directly interact with instance variables or methods.
A static variable in Java acts like a global variable within a class, consistent across all objects.
🔥Key Features of Static Methods:
Static Variables, Methods, and Blocks in Java:
- Can be called using the class name (
ClassName.methodName()
). - Can only access static data members.
- Cannot use
this
orsuper
keywords. - Cannot directly call non-static methods.
Syntax:
javaclass ClassName {
static void methodName() {
// Code here
}
}
Example Usage:
javaclass MathUtil {
static int square(int num) {
return num * num;
}
}
public class Demo {
public static void main(String[] args) {
System.out.println("Square of 5: " + MathUtil.square(5)); // Output: Square of 5: 25
}
}
🔥What is a Static Block in Java?
A static block in Java is a block of code inside a class that runs once when the class is loaded into memory. It is typically used for static variable initialization.
🔥Key Features of Static Blocks:
- Executed only once when the class is loaded.
- Runs before any constructors or static methods.
- Used for initializing static variables.
Syntax:
javaclass ClassName {
static {
// Initialization code
}
}
Example Usage:
javaclass Config {
static int maxUsers;
static {
maxUsers = 100;
System.out.println("Static block executed! Max users set to " + maxUsers);
}
}
public class Demo {
public static void main(String[] args) {
System.out.println("Max users: " + Config.maxUsers);
}
}
Output:
bashStatic block executed! Max users set to 100
Max users: 100
🔥Static Variables Insights on Static in Java:-
- Why Use Static in Java? Static variables reduce memory usage by storing data at the class level.
- Best Practices for Static Methods: Use them for utility functions like mathematical operations and configuration settings.
- Static Block vs Constructor: A constructor is invoked when an object is created, whereas a static block runs once when the class is loaded.
- Performance Considerations: Using static variables excessively can lead to memory leaks if they are not cleared properly.
By understanding static variables, methods, and blocks, developers can write optimized and efficient Java programs. 🚀